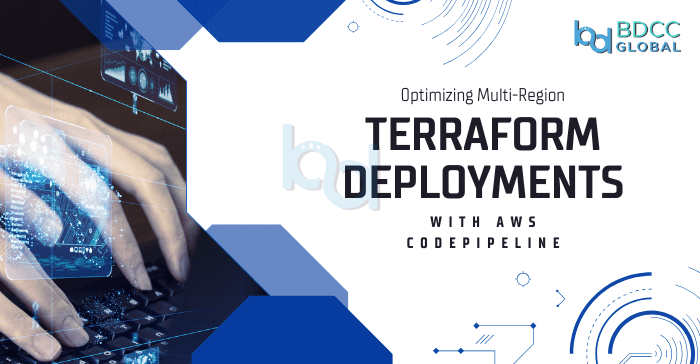
What happens when a cloud outage disrupts your operations, or global users experience slow performance? Multi-region Terraform deployment in AWS solves these challenges by ensuring high availability, disaster recovery, and optimized latency.
However, managing infrastructure across multiple AWS regions is complex. Terraform’s Infrastructure as Code (IaC) simplifies provisioning, but automation is key to consistency and efficiency. Terraform AWS CodePipeline streamlines workflows, automating deployments and minimizing errors.
In this guide, we’ll explore best practices for multi-region Terraform deployment using Terraform AWS CodePipeline, covering efficient code structuring, secure state management, automation strategies, and expert consulting insights. Let’s get started.
You might also want to know about effortless cloud transformation with Terraform consulting.
Multi-Region Terraform Deployment: Key Concepts
Deploying infrastructure across multiple AWS regions enhances availability, compliance, and performance while introducing challenges such as state management, dependency handling, and automation. Terraform provides tools to simplify multi-region Terraform deployment while maintaining consistency and efficiency.
Understanding Multi-Region Architecture
A multi-region Terraform deployment ensures resilience and business continuity by distributing workloads across regions, reducing latency, and improving redundancy.
Key benefits include:
- High Availability & Disaster Recovery – Maintains uptime even if one region fails.
- Compliance & Data Residency – Meets regulatory requirements (e.g., GDPR, HIPAA).
- Performance Optimization – Reduces latency for global applications.
- Redundancy & Fault Tolerance – Ensures seamless failover between AWS regions.
Terraform Workspaces for Multi-Region Deployments
Terraform workspaces help manage separate environments (e.g., development, staging, production) within the same Terraform codebase. Workspaces allow teams to isolate deployments while keeping configurations flexible.
How Terraform Workspaces Manage Multi-Region Deployments
By using Terraform workspaces, teams can:
- Isolate deployments for different environments while using the same infrastructure code.
- Keep environment-specific configurations without duplicating Terraform modules.
- Automate infrastructure provisioning across multiple regions efficiently.
Example Workflow:
1. Define a Terraform provider block for multiple regions:
provider "aws" {
region = var.aws_region
}
2. Use workspaces to switch between regions dynamically:
terraform workspace new us-east-1
terraform workspace new eu-west-1
terraform workspace select us-east-1
3. Deploy region-specific infrastructure using a variable-based approach:
variable "aws_region" {
default = "us-east-1"
}
Pros and Cons of Using Workspaces for Multi-Region Deployments
Pros | Cons |
---|---|
Simplifies multi-region Terraform deployment by avoiding duplicate code. | Workspaces primarily help with environmental isolation, but they do not natively handle state separation across regions. |
Reduces duplication of infrastructure code. | Complex deployments may require separate backend configurations for state storage. |
Enables faster switching between environments. | Not ideal for use cases that require multiple state files for each region instead of a single workspace. |
For large-scale multi-region deployments, teams often combine workspaces with separate Terraform state backends to enhance isolation and state management.
State Management in Multi-Region Deployments
A multi-region Terraform deployment relies on effective state management to track deployed resources and ensure consistency. Terraform state files should be stored securely to avoid corruption and misconfigurations.
In a multi-region deployment, managing Terraform state properly is critical to:
- Ensuring consistency across regions – Terraform must track which resources exist in each region.
- Preventing state corruption – Multiple team members working in different regions can introduce conflicts in shared state files.
- Facilitating team collaboration – A centralized state management approach enables teams to work seamlessly across multiple environments.
Best Practices for Multi-Region State Management
Use a Remote State Backend:
- Use Amazon S3 with DynamoDB locking for secure and collaborative state management. Store separate state files for each AWS region. Implement S3 versioning for backup and recovery.
- Example backend configuration
terraform {
backend "s3" {
bucket = "my-terraform-state"
key = "multi-region/terraform.tfstate"
region = "us-east-1"
encrypt = true
dynamodb_table = "terraform-lock"
}
}
Separate State Files by Region:
- Store state files in different S3 prefixes for each AWS region
s3://my-terraform-state/us-east-1/terraform.tfstate
s3://my-terraform-state/eu-west-1/terraform.tfstate
Leverage Terraform Workspaces for Better Isolation
- Combine workspaces with separate remote state configurations for full regional isolation.
Implement State Versioning and Backups
- Enable S3 versioning to restore state files in case of accidental corruption.
Cross-Region Dependencies
Multi-region deployments often require resources in one region to communicate with resources in another. Managing these cross-region dependencies efficiently is essential for maintaining security, performance, and availability.
Common Cross-Region Dependencies:
- VPC Peering – Direct network connectivity between VPCs in different AWS regions.
- AWS Transit Gateway – A scalable alternative to VPC peering for managing cross-region networking at scale.
- Cross-Region Replication (CRR) – Used for services like S3 buckets and DynamoDB global tables to replicate data across regions.
- IAM Role Trust Relationships – Configuring IAM roles to allow cross-region service access.
Best Practices for Managing Cross-Region Dependencies
Use AWS Transit Gateway for Scalability
- Transit Gateway simplifies multi-region networking compared to multiple VPC peerings.
Leverage Cross-Region Replication for Data Synchronization
- Enable S3 CRR to replicate storage across AWS regions.
- Use DynamoDB Global Tables for real-time multi-region database synchronization.
Manage IAM Roles with Region-Specific Policies
- Ensure that cross-region IAM permissions are restricted to required services.
Minimize Latency with Regional Endpoints
- Use Route 53 latency-based routing to direct traffic to the closest AWS region.
How to Integrate Terraform with AWS CodePipeline?
Terraform AWS CodePipeline automates deployments, ensuring consistency and reducing human error. It follows a three-stage workflow: Source, Build, and Deploy.
Overview of AWS CodePipeline
WS CodePipeline is a fully managed CI/CD service that automates software and infrastructure deployments. It operates through a series of stages, ensuring changes move through a structured workflow before being applied. The three key stages of a CodePipeline deployment for Terraform include:
- Source Stage: Retrieves Terraform code from a repository (e.g., AWS CodeCommit, GitHub, or Bitbucket).
- Build Stage: Uses AWS CodeBuild to validate and test Terraform configurations. Runs Terraform commands (terraform init, terraform plan) to check for potential issues.
- Deploy Stage: Executes terraform apply to deploy infrastructure across AWS regions. Implements manual approval steps if required.
Benefits of Using CodePipeline for Terraform Deployments
- Automated Deployment Workflow – Reduces manual intervention, ensuring consistency.
- Version Control Integration – Tracks changes, making rollback and auditing easier.
- Parallel and Sequential Deployment Support – Enables multi-region deployment strategies.
- Security & Governance – Implements approval workflows and permissions for controlled deployments.
Setting Up a Terraform Pipeline in AWS CodePipeline
This section outlines a step-by-step approach to integrating Terraform with AWS CodePipeline.
Source Stage: Connecting to a Git Repository
Terraform configurations must be stored in a version control system such as AWS CodeCommit, GitHub, or Bitbucket.
{
"name": "Source",
"actions": [
{
"name": "SourceAction",
"actionTypeId": {
"category": "Source",
"owner": "AWS",
"provider": "CodeCommit",
"version": "1"
},
"configuration": {
"RepositoryName": "terraform-repo",
"BranchName": "main"
}
}
]
}
Build Stage: Running Terraform Commands
AWS CodeBuild acts as the execution engine for Terraform within CodePipeline. It runs validation checks and Terraform plan commands.
Buildspec.yml for Terraform:
version: 0.2
phases:
install:
commands:
- echo "Installing Terraform..."
- curl -o terraform.zip https://releases.hashicorp.com/terraform/1.4.6/terraform_1.4.6_linux_amd64.zip
- unzip terraform.zip
- mv terraform /usr/local/bin/
pre_build:
commands:
- echo "Initializing Terraform..."
- terraform init
build:
commands:
- echo "Validating Terraform Configuration..."
- terraform validate
- terraform plan -out=tf
plan post_build:
commands:
- echo "Terraform plan completed"
Deploy Stage: Applying Terraform Configuration
Once the plan is validated, the deploy stage applies the Terraform configuration to provision AWS resources.
{
"name": "Deploy",
"actions": [
{
"name": "DeployAction",
"actionTypeId": {
"category": "Deploy",
"owner": "AWS",
"provider": "CodeBuild",
"version": "1"
},
"configuration": {
"ProjectName": "TerraformDeploy"
}
}
]
}
Best Practices for Deploy Stage:
- Use manual approval steps before applying Terraform changes.
- Implement rollback strategies in case of failed deployments.
- Store Terraform logs in AWS CloudWatch for debugging.
Best Practices for Multi-Region Terraform Deployments
To ensure smooth multi-region deployments, follow these best practices:
Infrastructure as Code Best Practices
- Use Terraform Modules – Modularize code to improve reusability across regions.
- Leverage Variables & Outputs – Pass region-specific values dynamically.
- Version Control & Code Reviews – Implement pull request approvals for Terraform changes.
Security and Compliance
- Secure Terraform State – Store in S3 with encryption; enable DynamoDB state locking.
- Limit IAM Permissions – Follow least privilege access for Terraform execution roles.
- Ensure Compliance – Implement region-based data residency policies (e.g., GDPR, HIPAA).
Cost Optimization
- Use Spot & Reserved Instances – Reduce EC2 costs across multiple regions.
- Monitor AWS Budgets – Set cost alerts for multi-region deployments.
- Avoid Unused Resources – Implement automated cleanup for unused Terraform resources.
Monitoring and Logging
- Enable AWS CloudWatch & X-Ray – Track Terraform deployment performance.
- Store Terraform Logs – Use S3 and CloudTrail for audit logs.
Disaster Recovery and Rollback Strategies
- Use Terraform Plan Before Apply – Identify potential failures in advance.
- Enable Rollbacks – Implement manual approval steps before infrastructure updates.
- Automate Backup Strategies – Use RDS Multi-AZ and cross-region replication for failover.
Final Recommendations
A successful multi-region Terraform deployment requires strategic planning, automation, and security best practices. By integrating Terraform AWS CodePipeline, organizations can automate deployments and maintain scalable, resilient infrastructure.
Next Steps:
- Implement remote state management using S3 and DynamoDB locking for secure and consistent multi-region deployments.
- Automate Terraform pipelines with AWS CodePipeline, integrating Git-based workflows for continuous deployment.
- Leverage best practices for Terraform deployment, including code modularization, security hardening, and compliance monitoring.
- Evaluate top Terraform consulting services for expert guidance on optimizing multi-region deployments at scale.
By following these best practices for multi-region Terraform deployment, organizations can achieve highly scalable, resilient, and cost-effective infrastructure automation.
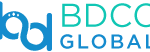
BDCC
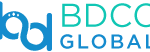
Latest posts by BDCC (see all)
- Enhancing Security Posture with Azure’s AI-Driven Threat Detection - April 15, 2025
- Why Golang is Becoming the Go-To Language for DevOps Engineers - April 11, 2025
- Azure Arc: Extending Azure Services to Hybrid and Multi-Cloud Environments - April 8, 2025