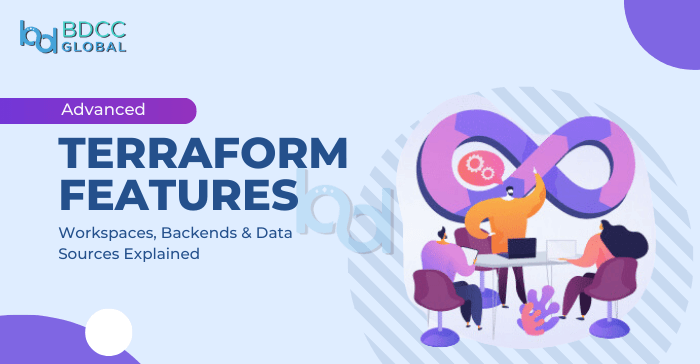
Have you ever wondered how to manage multiple environments like development, staging, and production without mixing them up? Or how do teams ensure that everyone is working simultaneously with the same setup without accidental overwrites? What if you could reuse parts of your infrastructure across projects instead of starting from scratch every time?
These are common challenges in managing cloud resources, and terraform, an Infrastructure-as-Code (IaC) tool, offers solutions. In this guide, we’ll introduce you to some of Terraform’s advanced features designed to make your life easier. By the end, you’ll understand how these features can help you manage cloud infrastructure with ease, even if you’re just starting out.
What is Terraform?
It is a tool, made by HashiCorp, that lets you define, deploy, and manage infrastructure using code. Instead of traditionally setting up servers, and other cloud resources, you can write simple configuration files that Terraform uses to automate the process.
What Can You Do with Terraform?
- Set Up Infrastructure Quickly: Deploy virtual machines, databases, and networks with just a few lines of code.
- Track Infrastructure Changes: Terraform keeps track of your resources in a state file, ensuring updates are consistent and predictable.
- Integrate Across Clouds: Manage resources across multiple providers (e.g., AWS, Azure, GCP) from a single tool.
What is the Purpose of Terraform?
There are multiple reasons capable enough to convince you to use Terraform, some of them are written below:
- Multi-Cloud Deployment: It can support various cloud providers and make it possible to manage resources within different platforms.
- Version Control: With the help of Terraform, you can make different versions of the infrastructure, share it and collaborate.
- Less Human Errors: By defining infrastructure as code, the change of errors minimize.
- More Efficiency: Terraform automates the management to save time and resources.
Why Should you Go Beyond the Basic Features?
While Terraform’s basic features are sufficient for small-scale projects, you might need advanced features for complex infrastructures. As your infrastructure grows, handeling various environments can become difficult. Therefore, advanced features can help you handle the complexity easily.
Similarly, in a team setting, it can be very critical to maintain, and update shared Terraform safely. However, with a backend you can safely store and lock the states in remote locations.
Infrastructures usually depend on existing resources, like AMIs or existing networks. But with data sources, you can integrate external resources easily into your configurations.
The Power of Advanced Features
Terraform’s advanced features unlock powerful capabilities to address these challenges:
- Workspaces: Help manage separate environments using the same codebase, ensuring consistency and reducing duplication.
- Backends: Centralize Terraform state storage, enabling secure sharing, state locking, and secure collab across teams.
- Data Sources: Fetch existing infrastructure data dynamically, integrating pre-existing resources into your Terraform-managed ecosystem.
What are Workspaces in Terraform?
Workspaces in Terraform provide a mechanism to maintain isolated state environments in a single configuration directory. This feature allows you to handle different versions or instances of your infrastructure without duplicating code. It is particularly useful for managing numerous environments, i.e., dev, staging, and production.
It is essentially a separate state file. Each workspace has its own state, meaning the resources it tracks and manages are unique to that workspace. Terraform usually uses a single workspace called default. However, it is your choice to set up numerous workspaces to handle various settings or use cases.
Key features of Workspaces:
1. State Isolation:
a. Each workspace maintains a separate state file, ensuring isolation between environments.
b. Alterations in one workspace do not affect resources in other workspaces.
2. Default and Custom Workspaces:
a. Default Workspace: Automatically created when you initialize a Terraform project. It’s suitable for single environment use cases.
b. Custom Workspaces: You can create and switch between workspaces as needed, such as dev, staging, prod.
3. Environment-Specific Resource Management:
a. Using workspaces, you can deploy the same configuration with variations tailored for different environments.
CLI Commands
Terraform provides simple commands to manage workspaces:
1. Create a New Workspace:
terraform workspace new
Example:
Terraform workspace new dev
2. List Existing Workspaces:
terraform workspace list
Output:
* default
Dev
staging
The current workspace is marked with an asterisk (*).
3.Switch Between Workspaces:
terraform workspace select <workspace_name>
4.Show Current Workspace:
terraform workspace show
5.Delete a Workspace (Note: You cannot delete the default workspace):
terraform workspace delete <workspace_name>
Use Case: Managing Multiple Environments with Workspaces
When managing cloud infrastructure, it’s common to have multiple environments to ensure smooth development and deployment processes. These environments are often segmented into Development (dev), Staging (staging), and Production (prod) to serve specific purposes. Terraform’s workspaces offer an elegant solution for managing these environments without duplicating configuration files or creating separate directories.
Why Multiple Environments?
1. Development (dev):
a. Purpose: A sandbox for developers to test new configurations and features without affecting the live environment.
b. Characteristics:
i. Small-scale resources, such as smaller instance sizes (e.g., t2.micro).
ii. Frequent changes and deployments.
iii. Minimal cost, as it’s only used for testing.
Example: Multi-Environment Management provider "aws" { region = "us-west-2" } resource "aws_s3_bucket" "example" { bucket = "my-bucket-${terraform.workspace}" acl = "private" tags = { Name = "MyBucket-${terraform.workspace}" Environment = terraform.workspace } }
Backends in Terraform
A Terraform backend defines where and how the state file is stored. The state file is an important part of Terraform’s functionality – it tracks the real-world resources Terraform manages. Without proper state management, Terraform cannot determine what exists, what needs to be created, or what needs to be updated or deleted.
By default, Terraform keeps the state file stored locally on your system. However, as projects grow and teams collaborate, remote backends become essential for:
- Sharing state across team members.
- Locking state to prevent simultaneous updates that can cause inconsistencies.
- Centralizing state storage for better reliability and access control.
Types of Backends
1. Local Backend:
- Keep the state file on your local disk.
- Suitable for single-user projects or experimentation.
- Default behavior if no backend is configured.
2. Remote Backends:
- Place the state file in a remote location, i.e., cloud storage & Terraform Cloud.
- Support state locking and team collaboration.
- Examples: Amazon S3, Azure Blob Storage, Google Cloud Storage, HashiCorp Consul, Terraform Cloud, etc.
Example: S3 Backend with State Locking terraform { backend "s3" { bucket = "my-terraform-state" key = "state/terraform.tfstate" region = "us-west-2" encrypt = true dynamodb_table = "terraform-lock-table" } }
Key Features of Backends
State Locking:
Prevents multiple users from applying changes simultaneously, which could corrupt the state file. Some backends (like S3) require an additional service (e.g., DynamoDB) to handle locking.
Centralization:
Remote backends provide a single source of truth, ensuring all team members work in the same state.
Collaboration:
Multiple users can safely access and update the infrastructure using a shared backend.
Security:
Remote backends often support encryption (e.g., S3 with server-side encryption) to secure sensitive state data.
Configuring a Backend
Example: Configuring an S3 Backend with State Locking
The following example demonstrates how to use an S3 backend for state storage with DynamoDB for state locking:
terraform { backend "s3" { bucket = "my-terraform-state" key = "path/to/my/state" region = "us-west-2" encrypt = true dynamodb_table = "terraform-lock-table" } }
Explanation:
- bucket: Specifies the S3 bucket to store the state file.
- key: Defines the road map to the state file in the bucket.
- region: Sets the AWS region for the S3 bucket.
- encrypt: Enables server-side encryption for the state file.
- dynamodb_table: Highlights the DynamoDB table for state locking.
DynamoDB Table Setup:
Before using state locking, create a DynamoDB table:
Aws dynamodb create-table \
— table-name terraform-locks \
— attribute-definitions attributeName=LockID,AttributeType=S \
— key-schema AttributeName=LockID,keyType=HASH \
— provisioned-throughput ReadCapacityUnits=5,WriteCapacityUnits=5
CLI Commands for Backends
- Initialize a Backend: After configuring a backend, run: “terraform init”
This command:
- Initializes the backend configuration.
- Migrates the state file (if needed).
2. Change Backend Configuration: If you modify the backend configuration, Terraform will prompt to confirm state migration.
3. Validate Backend: Test backend configuration using: “terraform validate”
Use Case
Scenario:
A team of developers is working on a shared infrastructure project. They need to:
- Collaborate safely without overwriting each other’s changes.
- Make sure that the state file is safely kept and backed up.
- Lock the state during updates to avoid conflicts.
Solution:
- Configure a remote backend (e.g., S3) with state locking using DynamoDB.
- Developers initialize Terraform with terraform init and work on the shared infrastructure.
Benefits:
- A single source of truth for the state file.
- Simultaneous access with safe locking.
- Encryption to secure sensitive infrastructure details.
Backend Comparison Table
Backend Type | State Locking | Encryption | Best For |
---|---|---|---|
Local | No | No | Single-user projects or testing |
S3 + DynamoDB | Yes | Yes | Teams need scalable remote storage |
Azure Blob | Yes | Yes | Microsoft Azure environments. |
Google Cloud | Yes | Yes | Google Cloud infrastructure. |
Terraform Cloud | Yes | Yes | Full-featured collaboration |
By leveraging backends, you can scale Terraform for team-based projects, improve security, and ensure consistent state management for reliable infrastructure provisioning.
Data Sources in Terraform
Data sources in Terraform are used to gather information about resources or external data. Unlike resources, which define infrastructure components to be created, data sources are read-only and retrieve data that can then be used in your configuration.
Why Use Data Sources?
- Access details about existing infrastructure without duplicating resource creation.
- Integrate Terraform with pre-existing resources managed outside Terraform (e.g., an existing VPC, security group, or AMI).
- Dynamically reference data that changes over time, such as the latest AMI ID.
Key Features of Data Sources
Read-Only
Data sources do not modify resources; they only fetch information. Example: Retrieve the ID of the most recent Amazon Machine Image (AMI).
Dynamic Inputs
Enable dynamic referencing of resources. Example: Fetch a VPC ID based on tags and use it in a subnet configuration.
Integration
Seamlessly integrate Terraform with existing infrastructure and external data sources like remote state files or APIs.
Common Data Source Examples
Example 1: Fetching an AWS AMI You can use a data source to retrieve the most recent AMI matching specific criteria. data "aws_ami" "example" { most_recent = true owners = ["self"] filter { name = "name" values = ["my-custom-ami-*"] } } resource "aws_instance" "example" { ami = data.aws_ami.example.id instance_type = "t2.micro" }
Explanation:
- The aws_ami data source fetches the latest AMI that matches the criteria.
- The AMI ID is dynamically passed to the aws_instance resource.
Example 2: Using Remote State with Terraform Cloud or S3
Data sources can fetch the output values of another Terraform configuration’s remote state.
Data “terraform_remote_state” “example” { Backend = ‘s3” Config = { Bucket = “my-state-bucket" Key - “state.tfstate” Region = “us-west-2" } } Output “vpc_id”{ Value = data.etrraform_remote_state.example.outputs.vpc_id }
Explanation:
- This configuration fetches the output vpc_id from a remote state file stored in an S3 bucket.
- This enables reuse of infrastructure components (like a VPC) across multiple projects.
Use Case
- Scenario: You have a pre-existing VPC and want to deploy new resources into it without recreating the VPC in Terraform.
- Solution: Use a data source to fetch the VPC’s ID and pass it to the new resources:
Data “aws_vpc” “default” { Default = true #Fetch the default VPC in the account } Resource “aws_subnet” “example” { Vpc_id = data.aws_vpc.default.id Cidr_block = “10.0.1.0/24” Availability_zone = “us-west-2a" }
Combining Workspaces, Backends, and Data Sources
Combining these features – Workspaces, Backends, and Data Sources – makes Terraform:
- Scalability: Handle multiple environments (dev, staging, prod) without duplicating code.
- Flexibility: Dynamically fetch existing infrastructure details (e.g., AMI IDs, VPCs) for integration.
- Collaboration: Use centralized and secure state storage for team-based workflows.
Example Workflow
- Workspaces for Environments:
- Create separate workspaces for dev, staging, and prod.
- Use workspace variables to adjust configurations dynamically.
2. Backends for State Management:
- Store state in a remote backend (e.g., S3) for centralized access and locking.
3. Data Sources for Dynamic Inputs:
- Fetch existing infrastructure details (e.g., AMI IDs or VPC IDs) using data sources.
Example Configuration: Terraform { Backend “s3” { bucket = “my-terraform-states' Key = “env/${terraform.workspace}/terraform.tfstate” Region = “us-east-1" } } #Fetch the most recent AMI Data “aws_ami” “latest” { Most-recent = true Owners = [“self”] } #Fetch a pre-existing VPC Data “aws_vpc” “default” { Default = true } #Launch an EC2 instance in the dafult VPC Resource “aws_instance” “web” { Ami = data.aws_ami.latest.id Instance_type = “t2.micro” Subnet_id = data.aws_ami.latest.id Tags = { Environment = terraform.worksppace } }
Key Features in This Configuration:
Workspaces:
- The backend uses ${terraform.workspace} to dynamically store state files for every environment.
- The tags block includes the workspace name to tag resources appropriately.
Backends:
- Centralized state storage in S3, ensuring consistency and team collaboration.
- State locking with DynamoDB (if configured) prevents conflicts.
Data Sources:
- Fetches the latest AMI for the EC2 instance.
- Retrieves the default VPC ID for deploying the instance.
Benefits of Combining Terraform Workspaces, Backends, and Data Sources
Environment-Specific Configurations
Using Workspaces, you can manage different environments like dev, staging, and prod without creating separate Terraform projects. Each workspace has its own state file, so your configurations stay clean and organized, and you avoid mistakes like mixing up environments.
Example:
- Deploy a test database in the dev workspace.
- Deploy the same configuration with production settings in the prod workspace. This helps ensure consistency across environments while keeping them isolated.
Reliable State Management
Backends allow you to store Terraform’s state file in a central and secure location, like an S3 bucket or Terraform Cloud. This makes it easier for teams to collaborate on the same infrastructure.
- State Locking: Prevents multiple people from accidentally updating the infrastructure at the same time.
- Centralized Access: Everyone on the team works with the same state file, so there are no conflicts or outdated configurations.
Example:
A team of developers can share a single S3-backed state file and use DynamoDB for state locking. This ensures no one overwrites the state while another team member is applying changes.
Dynamic Resource Integration
Data Sources lets you pull information about existing infrastructure instead of recreating it in Terraform. This reduces duplication and ensures Terraform works well with resources created outside of it.
Example:
- Fetch the ID of an existing VPC to deploy a new subnet.
- Dynamically retrieve the latest AMI ID to ensure EC2 instances always use up-to-date images.
By referencing existing infrastructure, you save time, avoid errors, and keep your configurations lightweight.
Conclusion
Managing infrastructure efficiently, securely, and collaboratively is crucial for any team working on cloud-based solutions. Terraform provides the tools to achieve this, but its advanced features – Workspaces, Backends, and Data Sources – take your infrastructure management to the next level.
Terraform’s advanced features empower you to handle any infrastructure challenge with confidence. Whether you’re managing a single environment or a complex multi-cloud setup, these tools make your workflow efficient, secure, and scalable. Start small, explore these features, and watch your infrastructure management evolve.
FAQs
What is the main purpose of Terraform Workspaces?
Terraform Workspaces lets you isolate environments (like dev, staging, and prod) within a seperate configuration directory. Each workspace has its own state file, keeping environments separate while sharing the same codebase.
Why do I need Backends in Terraform?
Backends are essential for:
- Centralizing the Terraform state file, keeping it reachable to every team member.
- Enabling state locking to prevent simultaneous updates.
- Ensuring secure storage with encryption options.
Can I use Workspaces, Backends, and Data Sources together?
Yes! These features are made to favor each other:
- Use Workspaces to isolate environments.
- Configure a Backend to centralize and secure your state files.
- Use Data Sources to dynamically fetch details about existing infrastructure.
How do Backends handle state locking?
Certain backends (e.g., S3 with DynamoDB) support state locking. This prevents multiple users from applying changes simultaneously, avoiding state corruption or conflicts.
Is it necessary to use advanced features for small projects?
For small, single-user projects, these features may not be necessary. However, as your project grows or involves collaboration, using Workspaces, Backends, and Data Sources becomes critical to ensure scalability and security.
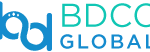
BDCC
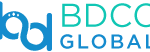
Latest posts by BDCC (see all)
- Cloud computing in healthcare: Uses and benefits - June 23, 2025
- What are the Best Practices for Terraform File Structure? - June 10, 2025
- From Monolith to Microservices: DevOps Strategies For a Smooth Transition - June 5, 2025